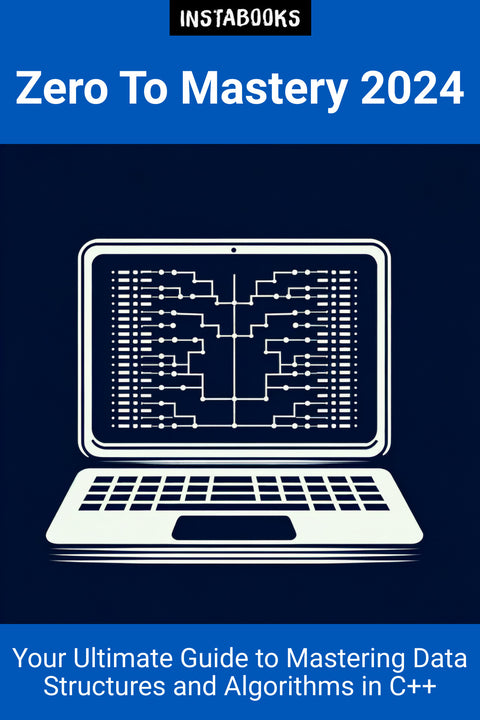
Instabooks AI (AI Author)
Zero To Mastery 2024
Your Ultimate Guide to Mastering Data Structures and Algorithms in C++
Premium AI Book - 200+ pages



Zero To Mastery 2024: Your Ultimate Guide to Mastering Data Structures and Algorithms in C++
Dive into the world of Data Structures and Algorithms (DSA) with Zero To Mastery 2024, your comprehensive source for mastering these essential concepts using C++. From beginners dipping their toes into the basics to experts refining their skills, this book provides a structured journey through the complexities of DSA in one of the most powerful programming languages in the world.
Explore the intricacies of arrays, linked lists, trees, and graphs as you progress through clear, methodical explanations. Delve into sorting and searching algorithms, mastering techniques from the simple to the most advanced. Every chapter is enriched with practical examples and exercises designed to solidify your understanding and enhance your problem-solving skills.
Unlock the full potential of C++ and its application to DSA with detailed walkthroughs of code and innovative problem-solving strategies. Discover optimizations and efficiency tips that will turn complex problems into simpler challenges. With Zero To Mastery 2024, you’ll not only learn, but you’ll master the algorithms and structures that form the backbone of computer science.
Designed to cater to all levels of experience, this book emphasizes clarity in explanation for beginners, while providing advanced readers with deep dives into complex theories. Whether you’re a student, a professional programmer, or a hobbyist, this guide will elevate your coding expertise, preparing you for today’s competitive tech landscape.
Join the ranks of proficient C++ programmers who understand the power and elegance of algorithms and data structures. Make Zero To Mastery 2024 your essential resource for learning, mastering, and applying DSA principles in real-world applications.
Table of Contents
1. Introduction to C++ and Basics of DSA- Getting Started with C++
- Understanding the Fundamentals of Data Structures
- Introduction to Algorithms
2. Working With Arrays and Linked Lists
- Arrays: Definition, Operation, and Use Cases
- Singly and Doubly Linked Lists
- Implementing Stacks and Queues
3. Mastering Trees and Graphs
- Understanding Trees and Their Types
- Graph Theory Basics
- Implementing Trees and Graphs in C++
4. Exploring Sorting Algorithms
- Overview of Sorting Algorithms
- Implementing Popular Sorting Algorithms
- Advanced Sorting Techniques
5. Diving Into Searching Algorithms
- Linear and Binary Search
- Graph Search Algorithms: BFS and DFS
- Application of Searching Algorithms
6. Advanced Data Structures
- Hash Tables and Hashing
- Priority Queues and Heaps
- Advanced Tree Structures: AVL and Red-Black Trees
7. Dynamic Programming and Greedy Algorithms
- Basics of Dynamic Programming
- Exploring Greedy Algorithms
- Combining DP and Greedy for Optimization
8. Graph Algorithms in Depth
- Shortest Path Algorithms
- Minimum Spanning Tree
- Network Flow and Its Applications
9. String Algorithms
- Basic String Processing
- String Matching Algorithms
- Advanced Techniques in String Algorithms
10. Computational Geometry
- Introduction to Computational Geometry
- Basic Algorithms and Applications
- Advanced Topics in Computational Geometry
11. Parallel and Distributed Algorithms
- Parallel Computing Basics
- Parallel Algorithms for DSA
- Distributed Algorithms and Their Applications
12. Final Thoughts and Advanced Topics
- The Future of Algorithms and Data Structures
- Preparing for Interviews and Competitions
- Where to Go from Here?
How This Book Was Generated
This book is the result of our advanced AI text generator, meticulously crafted to deliver not just information but meaningful insights. By leveraging our AI book generator, cutting-edge models, and real-time research, we ensure each page reflects the most current and reliable knowledge. Our AI processes vast data with unmatched precision, producing over 200 pages of coherent, authoritative content. This isn’t just a collection of facts—it’s a thoughtfully crafted narrative, shaped by our technology, that engages the mind and resonates with the reader, offering a deep, trustworthy exploration of the subject.
Satisfaction Guaranteed: Try It Risk-Free
We invite you to try it out for yourself, backed by our no-questions-asked money-back guarantee. If you're not completely satisfied, we'll refund your purchase—no strings attached.